How Developers Can Improve Their Debugging Techniques By Gustavo Woltmann
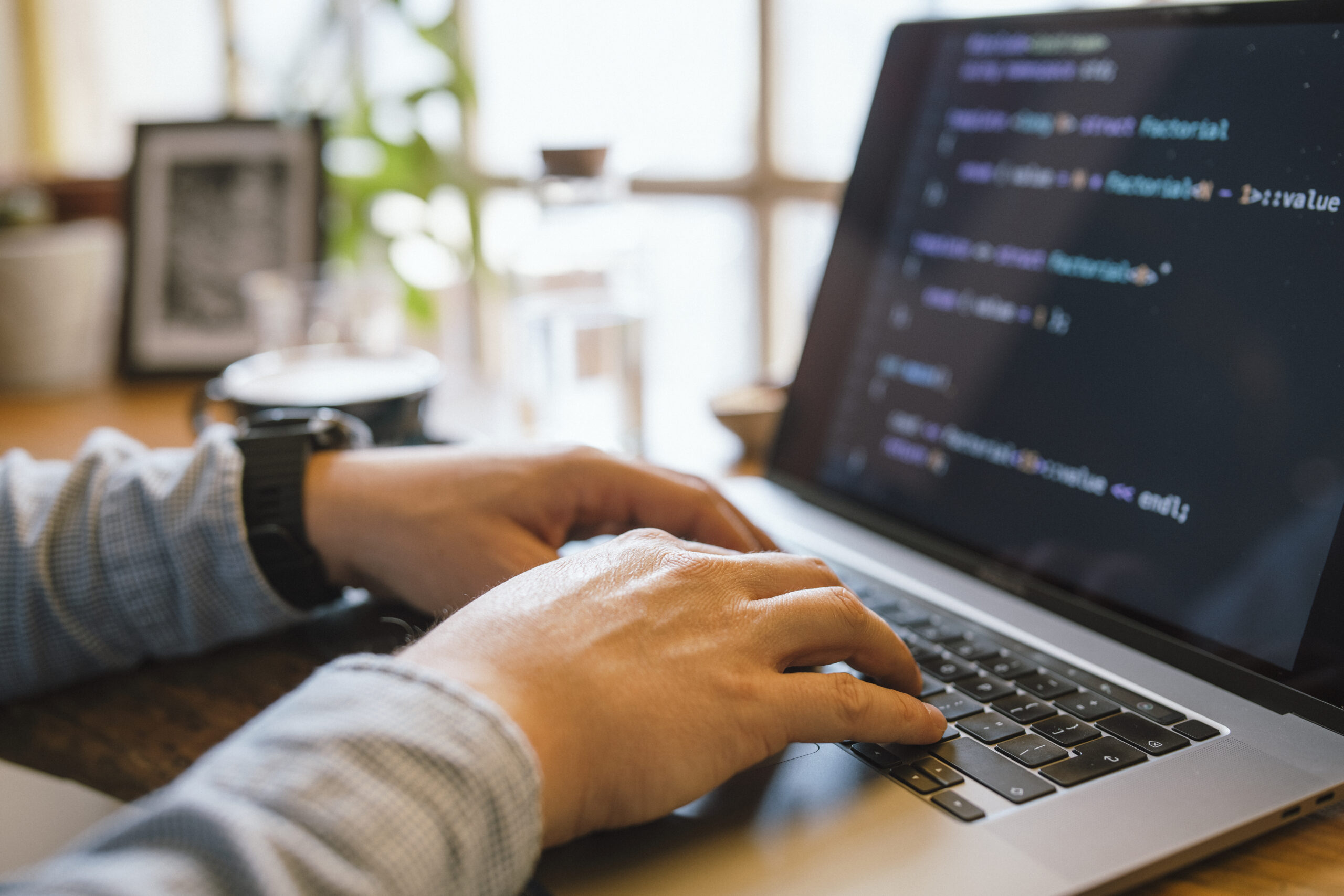
Debugging is Just about the most critical — however typically forgotten — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Understanding to Consider methodically to resolve troubles proficiently. Irrespective of whether you are a rookie or perhaps a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and substantially increase your productiveness. Allow me to share many techniques to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is 1 part of enhancement, recognizing the way to interact with it effectively all through execution is Similarly essential. Modern enhancement environments appear equipped with impressive debugging abilities — but numerous developers only scratch the surface of what these instruments can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by code line by line, and also modify code over the fly. When employed correctly, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform irritating UI difficulties into workable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about running processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic modifications.
Eventually, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not dropped in the dead of night. The higher you already know your instruments, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most vital — and infrequently missed — ways in productive debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders need to have to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise problems under which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar info, simulating similar consumer interactions, or mimicking process states. If The problem seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on certain working programs, browsers, or less than particular configurations. Employing applications like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging instruments extra effectively, test possible fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete problem — and that’s in which developers thrive.
Browse and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Erroneous. In lieu of looking at them as discouraging interruptions, developers ought to discover to take care of mistake messages as direct communications in the system. They frequently tell you exactly what happened, where it happened, and in some cases even why it transpired — if you understand how to interpret them.
Start off by reading through the concept very carefully and in comprehensive. A lot of developers, especially when underneath time strain, glance at the main line and quickly start off producing assumptions. But deeper within the error stack or logs may lie the accurate root trigger. Don’t just duplicate and paste mistake messages into engines like google — go through and realize them initial.
Crack the mistake down into pieces. Can it be a syntax error, a runtime exception, or perhaps a logic mistake? Will it position to a selected file and line range? What module or purpose induced it? These queries can manual your investigation and point you towards the accountable code.
It’s also helpful to understand the terminology of your programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and Studying to acknowledge these can greatly accelerate your debugging process.
Some problems are vague or generic, As well as in These conditions, it’s vital to look at the context in which the mistake occurred. Check out similar log entries, input values, and up to date variations in the codebase.
Don’t overlook compiler or linter warnings possibly. These frequently precede more substantial issues and provide hints about likely bugs.
Finally, error messages will not be your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, supporting you pinpoint difficulties faster, minimize debugging time, and turn into a more effective and assured developer.
Use Logging Correctly
Logging is one of the most potent equipment in a very developer’s debugging toolkit. When made use of effectively, it provides true-time insights into how an software behaves, serving to you have an understanding of what’s going on under the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic starts off with recognizing what to log and at what amount. Popular logging concentrations involve DEBUG, INFO, WARN, ERROR, and Lethal. Use DEBUG for detailed diagnostic info in the course of advancement, Data for standard functions (like productive commence-ups), WARN for possible problems that don’t crack the applying, Mistake for true issues, and FATAL in the event the procedure can’t go on.
Avoid flooding your logs with too much or irrelevant knowledge. Far too much logging can obscure important messages and slow down your system. Focus on vital functions, condition modifications, enter/output values, and demanding selection details as part of your code.
Format your log messages Evidently and persistently. Consist of context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you keep track of how variables evolve, what problems are satisfied, and what branches of logic are executed—all with out halting the program. They’re In particular worthwhile in production environments where by stepping by means of code isn’t feasible.
On top of that, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Eventually, smart logging is about stability and clarity. With a well-imagined-out logging method, you may reduce the time it will require to spot troubles, gain deeper visibility into your purposes, and improve the Over-all maintainability and reliability of your respective code.
Believe Similar to a Detective
Debugging is not only a complex task—it's a sort of investigation. To successfully establish and fix bugs, developers ought to approach the process just like a detective fixing a secret. This mindset aids stop working complicated troubles into workable parts and adhere to clues logically to uncover the root result in.
Start off by accumulating proof. Think about the symptoms of the trouble: error messages, incorrect output, or effectiveness challenges. Identical to a detective surveys a crime scene, collect just as much relevant info as you are able to without having jumping to conclusions. Use logs, test instances, and user reports to piece together a transparent photograph of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any adjustments not too long ago been created for the codebase? Has this situation transpired just before below comparable circumstances? The intention will be to slim down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a controlled natural environment. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes guide you nearer to the truth.
Pay back near interest to small information. Bugs frequently disguise inside the the very least predicted places—similar to a missing semicolon, an off-by-1 mistake, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty without having totally being familiar with it. Short term fixes may perhaps conceal the actual difficulty, just for it to resurface later.
And finally, keep notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated devices.
Write Exams
Composing checks is among the most effective methods to increase your debugging skills and All round progress efficiency. Exams not merely support capture bugs early but will also function a security net that gives you self-assurance when generating improvements towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint specifically the place and when a difficulty happens.
Begin with unit exams, which target particular person features or modules. These modest, isolated assessments can immediately expose whether a selected bit of logic is Doing the job as expected. When a test fails, you immediately know where to look, significantly reducing some time expended debugging. Device assessments are Specifically beneficial for catching regression bugs—troubles that reappear right after Formerly being preset.
Upcoming, combine integration exams and finish-to-end assessments into your workflow. These support make certain that numerous parts of your software operate with each other smoothly. They’re significantly valuable for catching bugs that take place in complicated systems with many elements or solutions interacting. If something breaks, your assessments can tell you which Element of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Believe critically regarding your code. To test a aspect appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of comprehending Obviously prospects to raised code construction and much less bugs.
When debugging a problem, producing a failing check that reproduces the bug is usually a powerful initial step. Once the take a look at fails consistently, it is possible to focus on repairing the bug and enjoy your test move when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable process—aiding you catch much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky situation, it’s quick to be immersed in the issue—watching your display screen for several hours, trying Remedy soon after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks will help you reset your head, cut down frustration, and often see The problem from a new viewpoint.
When you are far too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps start overlooking obvious errors or misreading code that you wrote just hours earlier. On this condition, your brain gets to be significantly less effective at issue-solving. A brief wander, a coffee break, or perhaps switching to a different task for ten–quarter-hour can refresh your emphasis. A lot of developers report discovering the root of a problem when they've taken the perfect time to disconnect, permitting their subconscious get the job done from the qualifications.
Breaks also aid avoid burnout, Particularly throughout longer debugging classes. Sitting down in front of a display, mentally stuck, is don't just unproductive but in addition draining. Stepping away allows you to return with renewed Electrical power and also a clearer way of thinking. You could possibly all of a sudden discover a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you right before.
In case you’re stuck, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to maneuver all around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than restricted deadlines, but it really truly leads to more rapidly and more effective debugging Eventually.
In short, getting breaks is not an indication of weak spot—it’s a smart tactic. It more info provides your Mind space to breathe, enhances your standpoint, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Master From Every Bug
Just about every bug you experience is much more than simply a temporary setback—It truly is a possibility to develop being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural difficulty, each one can educate you a thing valuable should you make an effort to mirror and examine what went Erroneous.
Start by asking oneself a number of critical questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with superior tactics like device tests, code reviews, or logging? The answers frequently reveal blind spots inside your workflow or knowing and allow you to Create more robust coding practices relocating forward.
Documenting bugs can be a fantastic routine. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring troubles or frequent blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, aiding Other people steer clear of the very same concern boosts team performance and cultivates a more robust Studying society.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital elements of your enhancement journey. All things considered, a few of the finest developers are usually not the ones who produce excellent code, but individuals that continually learn from their problems.
Eventually, Every single bug you fix adds a completely new layer in your talent set. So following time you squash a bug, take a second to replicate—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Strengthening your debugging skills will take time, observe, and patience — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The subsequent time you might be knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.